1 | # balanced-match
|
---|
2 |
|
---|
3 | Match balanced string pairs, like `{` and `}` or `<b>` and `</b>`. Supports regular expressions as well!
|
---|
4 |
|
---|
5 | [](http://travis-ci.org/juliangruber/balanced-match)
|
---|
6 | [](https://www.npmjs.org/package/balanced-match)
|
---|
7 |
|
---|
8 | [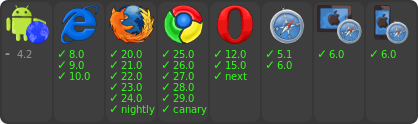](https://ci.testling.com/juliangruber/balanced-match)
|
---|
9 |
|
---|
10 | ## Example
|
---|
11 |
|
---|
12 | Get the first matching pair of braces:
|
---|
13 |
|
---|
14 | ```js
|
---|
15 | var balanced = require('balanced-match');
|
---|
16 |
|
---|
17 | console.log(balanced('{', '}', 'pre{in{nested}}post'));
|
---|
18 | console.log(balanced('{', '}', 'pre{first}between{second}post'));
|
---|
19 | console.log(balanced(/\s+\{\s+/, /\s+\}\s+/, 'pre { in{nest} } post'));
|
---|
20 | ```
|
---|
21 |
|
---|
22 | The matches are:
|
---|
23 |
|
---|
24 | ```bash
|
---|
25 | $ node example.js
|
---|
26 | { start: 3, end: 14, pre: 'pre', body: 'in{nested}', post: 'post' }
|
---|
27 | { start: 3,
|
---|
28 | end: 9,
|
---|
29 | pre: 'pre',
|
---|
30 | body: 'first',
|
---|
31 | post: 'between{second}post' }
|
---|
32 | { start: 3, end: 17, pre: 'pre', body: 'in{nest}', post: 'post' }
|
---|
33 | ```
|
---|
34 |
|
---|
35 | ## API
|
---|
36 |
|
---|
37 | ### var m = balanced(a, b, str)
|
---|
38 |
|
---|
39 | For the first non-nested matching pair of `a` and `b` in `str`, return an
|
---|
40 | object with those keys:
|
---|
41 |
|
---|
42 | * **start** the index of the first match of `a`
|
---|
43 | * **end** the index of the matching `b`
|
---|
44 | * **pre** the preamble, `a` and `b` not included
|
---|
45 | * **body** the match, `a` and `b` not included
|
---|
46 | * **post** the postscript, `a` and `b` not included
|
---|
47 |
|
---|
48 | If there's no match, `undefined` will be returned.
|
---|
49 |
|
---|
50 | If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `['{', 'a', '']` and `{a}}` will match `['', 'a', '}']`.
|
---|
51 |
|
---|
52 | ### var r = balanced.range(a, b, str)
|
---|
53 |
|
---|
54 | For the first non-nested matching pair of `a` and `b` in `str`, return an
|
---|
55 | array with indexes: `[ <a index>, <b index> ]`.
|
---|
56 |
|
---|
57 | If there's no match, `undefined` will be returned.
|
---|
58 |
|
---|
59 | If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `[ 1, 3 ]` and `{a}}` will match `[0, 2]`.
|
---|
60 |
|
---|
61 | ## Installation
|
---|
62 |
|
---|
63 | With [npm](https://npmjs.org) do:
|
---|
64 |
|
---|
65 | ```bash
|
---|
66 | npm install balanced-match
|
---|
67 | ```
|
---|
68 |
|
---|
69 | ## Security contact information
|
---|
70 |
|
---|
71 | To report a security vulnerability, please use the
|
---|
72 | [Tidelift security contact](https://tidelift.com/security).
|
---|
73 | Tidelift will coordinate the fix and disclosure.
|
---|
74 |
|
---|
75 | ## License
|
---|
76 |
|
---|
77 | (MIT)
|
---|
78 |
|
---|
79 | Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
|
---|
80 |
|
---|
81 | Permission is hereby granted, free of charge, to any person obtaining a copy of
|
---|
82 | this software and associated documentation files (the "Software"), to deal in
|
---|
83 | the Software without restriction, including without limitation the rights to
|
---|
84 | use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
|
---|
85 | of the Software, and to permit persons to whom the Software is furnished to do
|
---|
86 | so, subject to the following conditions:
|
---|
87 |
|
---|
88 | The above copyright notice and this permission notice shall be included in all
|
---|
89 | copies or substantial portions of the Software.
|
---|
90 |
|
---|
91 | THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
---|
92 | IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
---|
93 | FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
---|
94 | AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
---|
95 | LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
---|
96 | OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
---|
97 | SOFTWARE.
|
---|