[6a3a178] | 1 | # fastq
|
---|
| 2 |
|
---|
| 3 | ![ci][ci-url]
|
---|
| 4 | [![npm version][npm-badge]][npm-url]
|
---|
| 5 | [![Dependency Status][david-badge]][david-url]
|
---|
| 6 |
|
---|
| 7 | Fast, in memory work queue.
|
---|
| 8 |
|
---|
| 9 | Benchmarks (1 million tasks):
|
---|
| 10 |
|
---|
| 11 | * setImmediate: 812ms
|
---|
| 12 | * fastq: 854ms
|
---|
| 13 | * async.queue: 1298ms
|
---|
| 14 | * neoAsync.queue: 1249ms
|
---|
| 15 |
|
---|
| 16 | Obtained on node 12.16.1, on a dedicated server.
|
---|
| 17 |
|
---|
| 18 | If you need zero-overhead series function call, check out
|
---|
| 19 | [fastseries](http://npm.im/fastseries). For zero-overhead parallel
|
---|
| 20 | function call, check out [fastparallel](http://npm.im/fastparallel).
|
---|
| 21 |
|
---|
| 22 | [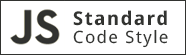](https://github.com/feross/standard)
|
---|
| 23 |
|
---|
| 24 | * <a href="#install">Installation</a>
|
---|
| 25 | * <a href="#usage">Usage</a>
|
---|
| 26 | * <a href="#api">API</a>
|
---|
| 27 | * <a href="#license">Licence & copyright</a>
|
---|
| 28 |
|
---|
| 29 | ## Install
|
---|
| 30 |
|
---|
| 31 | `npm i fastq --save`
|
---|
| 32 |
|
---|
| 33 | ## Usage (callback API)
|
---|
| 34 |
|
---|
| 35 | ```js
|
---|
| 36 | 'use strict'
|
---|
| 37 |
|
---|
| 38 | const queue = require('fastq')(worker, 1)
|
---|
| 39 |
|
---|
| 40 | queue.push(42, function (err, result) {
|
---|
| 41 | if (err) { throw err }
|
---|
| 42 | console.log('the result is', result)
|
---|
| 43 | })
|
---|
| 44 |
|
---|
| 45 | function worker (arg, cb) {
|
---|
| 46 | cb(null, arg * 2)
|
---|
| 47 | }
|
---|
| 48 | ```
|
---|
| 49 |
|
---|
| 50 | ## Usage (promise API)
|
---|
| 51 |
|
---|
| 52 | ```js
|
---|
| 53 | const queue = require('fastq').promise(worker, 1)
|
---|
| 54 |
|
---|
| 55 | async function worker (arg) {
|
---|
| 56 | return arg * 2
|
---|
| 57 | }
|
---|
| 58 |
|
---|
| 59 | async function run () {
|
---|
| 60 | const result = await queue.push(42)
|
---|
| 61 | console.log('the result is', result)
|
---|
| 62 | }
|
---|
| 63 |
|
---|
| 64 | run()
|
---|
| 65 | ```
|
---|
| 66 |
|
---|
| 67 | ### Setting "this"
|
---|
| 68 |
|
---|
| 69 | ```js
|
---|
| 70 | 'use strict'
|
---|
| 71 |
|
---|
| 72 | const that = { hello: 'world' }
|
---|
| 73 | const queue = require('fastq')(that, worker, 1)
|
---|
| 74 |
|
---|
| 75 | queue.push(42, function (err, result) {
|
---|
| 76 | if (err) { throw err }
|
---|
| 77 | console.log(this)
|
---|
| 78 | console.log('the result is', result)
|
---|
| 79 | })
|
---|
| 80 |
|
---|
| 81 | function worker (arg, cb) {
|
---|
| 82 | console.log(this)
|
---|
| 83 | cb(null, arg * 2)
|
---|
| 84 | }
|
---|
| 85 | ```
|
---|
| 86 |
|
---|
| 87 | ### Using with TypeScript (callback API)
|
---|
| 88 |
|
---|
| 89 | ```ts
|
---|
| 90 | 'use strict'
|
---|
| 91 |
|
---|
| 92 | import * as fastq from "fastq";
|
---|
| 93 | import type { queue, done } from "fastq";
|
---|
| 94 |
|
---|
| 95 | type Task = {
|
---|
| 96 | id: number
|
---|
| 97 | }
|
---|
| 98 |
|
---|
| 99 | const q: queue<Task> = fastq(worker, 1)
|
---|
| 100 |
|
---|
| 101 | q.push({ id: 42})
|
---|
| 102 |
|
---|
| 103 | function worker (arg: Task, cb: done) {
|
---|
| 104 | console.log(arg.id)
|
---|
| 105 | cb(null)
|
---|
| 106 | }
|
---|
| 107 | ```
|
---|
| 108 |
|
---|
| 109 | ### Using with TypeScript (promise API)
|
---|
| 110 |
|
---|
| 111 | ```ts
|
---|
| 112 | 'use strict'
|
---|
| 113 |
|
---|
| 114 | import * as fastq from "fastq";
|
---|
| 115 | import type { queueAsPromised } from "fastq";
|
---|
| 116 |
|
---|
| 117 | type Task = {
|
---|
| 118 | id: number
|
---|
| 119 | }
|
---|
| 120 |
|
---|
| 121 | const q: queueAsPromised<Task> = fastq.promise(asyncWorker, 1)
|
---|
| 122 |
|
---|
| 123 | q.push({ id: 42}).catch((err) => console.error(err))
|
---|
| 124 |
|
---|
| 125 | async function asyncWorker (arg: Task): Promise<void> {
|
---|
| 126 | // No need for a try-catch block, fastq handles errors automatically
|
---|
| 127 | console.log(arg.id)
|
---|
| 128 | }
|
---|
| 129 | ```
|
---|
| 130 |
|
---|
| 131 | ## API
|
---|
| 132 |
|
---|
| 133 | * <a href="#fastqueue"><code>fastqueue()</code></a>
|
---|
| 134 | * <a href="#push"><code>queue#<b>push()</b></code></a>
|
---|
| 135 | * <a href="#unshift"><code>queue#<b>unshift()</b></code></a>
|
---|
| 136 | * <a href="#pause"><code>queue#<b>pause()</b></code></a>
|
---|
| 137 | * <a href="#resume"><code>queue#<b>resume()</b></code></a>
|
---|
| 138 | * <a href="#idle"><code>queue#<b>idle()</b></code></a>
|
---|
| 139 | * <a href="#length"><code>queue#<b>length()</b></code></a>
|
---|
| 140 | * <a href="#getQueue"><code>queue#<b>getQueue()</b></code></a>
|
---|
| 141 | * <a href="#kill"><code>queue#<b>kill()</b></code></a>
|
---|
| 142 | * <a href="#killAndDrain"><code>queue#<b>killAndDrain()</b></code></a>
|
---|
| 143 | * <a href="#error"><code>queue#<b>error()</b></code></a>
|
---|
| 144 | * <a href="#concurrency"><code>queue#<b>concurrency</b></code></a>
|
---|
| 145 | * <a href="#drain"><code>queue#<b>drain</b></code></a>
|
---|
| 146 | * <a href="#empty"><code>queue#<b>empty</b></code></a>
|
---|
| 147 | * <a href="#saturated"><code>queue#<b>saturated</b></code></a>
|
---|
| 148 | * <a href="#promise"><code>fastqueue.promise()</code></a>
|
---|
| 149 |
|
---|
| 150 | -------------------------------------------------------
|
---|
| 151 | <a name="fastqueue"></a>
|
---|
| 152 | ### fastqueue([that], worker, concurrency)
|
---|
| 153 |
|
---|
| 154 | Creates a new queue.
|
---|
| 155 |
|
---|
| 156 | Arguments:
|
---|
| 157 |
|
---|
| 158 | * `that`, optional context of the `worker` function.
|
---|
| 159 | * `worker`, worker function, it would be called with `that` as `this`,
|
---|
| 160 | if that is specified.
|
---|
| 161 | * `concurrency`, number of concurrent tasks that could be executed in
|
---|
| 162 | parallel.
|
---|
| 163 |
|
---|
| 164 | -------------------------------------------------------
|
---|
| 165 | <a name="push"></a>
|
---|
| 166 | ### queue.push(task, done)
|
---|
| 167 |
|
---|
| 168 | Add a task at the end of the queue. `done(err, result)` will be called
|
---|
| 169 | when the task was processed.
|
---|
| 170 |
|
---|
| 171 | -------------------------------------------------------
|
---|
| 172 | <a name="unshift"></a>
|
---|
| 173 | ### queue.unshift(task, done)
|
---|
| 174 |
|
---|
| 175 | Add a task at the beginning of the queue. `done(err, result)` will be called
|
---|
| 176 | when the task was processed.
|
---|
| 177 |
|
---|
| 178 | -------------------------------------------------------
|
---|
| 179 | <a name="pause"></a>
|
---|
| 180 | ### queue.pause()
|
---|
| 181 |
|
---|
| 182 | Pause the processing of tasks. Currently worked tasks are not
|
---|
| 183 | stopped.
|
---|
| 184 |
|
---|
| 185 | -------------------------------------------------------
|
---|
| 186 | <a name="resume"></a>
|
---|
| 187 | ### queue.resume()
|
---|
| 188 |
|
---|
| 189 | Resume the processing of tasks.
|
---|
| 190 |
|
---|
| 191 | -------------------------------------------------------
|
---|
| 192 | <a name="idle"></a>
|
---|
| 193 | ### queue.idle()
|
---|
| 194 |
|
---|
| 195 | Returns `false` if there are tasks being processed or waiting to be processed.
|
---|
| 196 | `true` otherwise.
|
---|
| 197 |
|
---|
| 198 | -------------------------------------------------------
|
---|
| 199 | <a name="length"></a>
|
---|
| 200 | ### queue.length()
|
---|
| 201 |
|
---|
| 202 | Returns the number of tasks waiting to be processed (in the queue).
|
---|
| 203 |
|
---|
| 204 | -------------------------------------------------------
|
---|
| 205 | <a name="getQueue"></a>
|
---|
| 206 | ### queue.getQueue()
|
---|
| 207 |
|
---|
| 208 | Returns all the tasks be processed (in the queue). Returns empty array when there are no tasks
|
---|
| 209 |
|
---|
| 210 | -------------------------------------------------------
|
---|
| 211 | <a name="kill"></a>
|
---|
| 212 | ### queue.kill()
|
---|
| 213 |
|
---|
| 214 | Removes all tasks waiting to be processed, and reset `drain` to an empty
|
---|
| 215 | function.
|
---|
| 216 |
|
---|
| 217 | -------------------------------------------------------
|
---|
| 218 | <a name="killAndDrain"></a>
|
---|
| 219 | ### queue.killAndDrain()
|
---|
| 220 |
|
---|
| 221 | Same than `kill` but the `drain` function will be called before reset to empty.
|
---|
| 222 |
|
---|
| 223 | -------------------------------------------------------
|
---|
| 224 | <a name="error"></a>
|
---|
| 225 | ### queue.error(handler)
|
---|
| 226 |
|
---|
| 227 | Set a global error handler. `handler(err, task)` will be called
|
---|
| 228 | when any of the tasks return an error.
|
---|
| 229 |
|
---|
| 230 | -------------------------------------------------------
|
---|
| 231 | <a name="concurrency"></a>
|
---|
| 232 | ### queue.concurrency
|
---|
| 233 |
|
---|
| 234 | Property that returns the number of concurrent tasks that could be executed in
|
---|
| 235 | parallel. It can be altered at runtime.
|
---|
| 236 |
|
---|
| 237 | -------------------------------------------------------
|
---|
| 238 | <a name="drain"></a>
|
---|
| 239 | ### queue.drain
|
---|
| 240 |
|
---|
| 241 | Function that will be called when the last
|
---|
| 242 | item from the queue has been processed by a worker.
|
---|
| 243 | It can be altered at runtime.
|
---|
| 244 |
|
---|
| 245 | -------------------------------------------------------
|
---|
| 246 | <a name="empty"></a>
|
---|
| 247 | ### queue.empty
|
---|
| 248 |
|
---|
| 249 | Function that will be called when the last
|
---|
| 250 | item from the queue has been assigned to a worker.
|
---|
| 251 | It can be altered at runtime.
|
---|
| 252 |
|
---|
| 253 | -------------------------------------------------------
|
---|
| 254 | <a name="saturated"></a>
|
---|
| 255 | ### queue.saturated
|
---|
| 256 |
|
---|
| 257 | Function that will be called when the queue hits the concurrency
|
---|
| 258 | limit.
|
---|
| 259 | It can be altered at runtime.
|
---|
| 260 |
|
---|
| 261 | -------------------------------------------------------
|
---|
| 262 | <a name="promise"></a>
|
---|
| 263 | ### fastqueue.promise([that], worker(arg), concurrency)
|
---|
| 264 |
|
---|
| 265 | Creates a new queue with `Promise` apis. It also offers all the methods
|
---|
| 266 | and properties of the object returned by [`fastqueue`](#fastqueue) with the modified
|
---|
| 267 | [`push`](#pushPromise) and [`unshift`](#unshiftPromise) methods.
|
---|
| 268 |
|
---|
| 269 | Node v10+ is required to use the promisified version.
|
---|
| 270 |
|
---|
| 271 | Arguments:
|
---|
| 272 | * `that`, optional context of the `worker` function.
|
---|
| 273 | * `worker`, worker function, it would be called with `that` as `this`,
|
---|
| 274 | if that is specified. It MUST return a `Promise`.
|
---|
| 275 | * `concurrency`, number of concurrent tasks that could be executed in
|
---|
| 276 | parallel.
|
---|
| 277 |
|
---|
| 278 | <a name="pushPromise"></a>
|
---|
| 279 | #### queue.push(task) => Promise
|
---|
| 280 |
|
---|
| 281 | Add a task at the end of the queue. The returned `Promise` will be fulfilled (rejected)
|
---|
| 282 | when the task is completed successfully (unsuccessfully).
|
---|
| 283 |
|
---|
| 284 | This promise could be ignored as it will not lead to a `'unhandledRejection'`.
|
---|
| 285 |
|
---|
| 286 | <a name="unshiftPromise"></a>
|
---|
| 287 | #### queue.unshift(task) => Promise
|
---|
| 288 |
|
---|
| 289 | Add a task at the beginning of the queue. The returned `Promise` will be fulfilled (rejected)
|
---|
| 290 | when the task is completed successfully (unsuccessfully).
|
---|
| 291 |
|
---|
| 292 | This promise could be ignored as it will not lead to a `'unhandledRejection'`.
|
---|
| 293 |
|
---|
| 294 | <a name="drained"></a>
|
---|
| 295 | #### queue.drained() => Promise
|
---|
| 296 |
|
---|
| 297 | Wait for the queue to be drained. The returned `Promise` will be resolved when all tasks in the queue have been processed by a worker.
|
---|
| 298 |
|
---|
| 299 | This promise could be ignored as it will not lead to a `'unhandledRejection'`.
|
---|
| 300 |
|
---|
| 301 | ## License
|
---|
| 302 |
|
---|
| 303 | ISC
|
---|
| 304 |
|
---|
| 305 | [ci-url]: https://github.com/mcollina/fastq/workflows/ci/badge.svg
|
---|
| 306 | [npm-badge]: https://badge.fury.io/js/fastq.svg
|
---|
| 307 | [npm-url]: https://badge.fury.io/js/fastq
|
---|
| 308 | [david-badge]: https://david-dm.org/mcollina/fastq.svg
|
---|
| 309 | [david-url]: https://david-dm.org/mcollina/fastq
|
---|