[6a3a178] | 1 | <img width="75px" height="75px" align="right" alt="Inquirer Logo" src="https://raw.githubusercontent.com/SBoudrias/Inquirer.js/master/assets/inquirer_readme.svg?sanitize=true" title="Inquirer.js"/>
|
---|
| 2 |
|
---|
| 3 | # Inquirer.js
|
---|
| 4 |
|
---|
| 5 | [](http://badge.fury.io/js/inquirer)
|
---|
| 6 | [](http://travis-ci.org/SBoudrias/Inquirer.js)
|
---|
| 7 | [](https://codecov.io/gh/SBoudrias/Inquirer.js)
|
---|
| 8 | [](https://app.fossa.com/projects/git%2Bgithub.com%2FSBoudrias%2FInquirer.js?ref=badge_shield)
|
---|
| 9 |
|
---|
| 10 | A collection of common interactive command line user interfaces.
|
---|
| 11 |
|
---|
| 12 | ## Table of Contents
|
---|
| 13 |
|
---|
| 14 | 1. [Documentation](#documentation)
|
---|
| 15 | 1. [Installation](#installation)
|
---|
| 16 | 2. [Examples](#examples)
|
---|
| 17 | 3. [Methods](#methods)
|
---|
| 18 | 4. [Objects](#objects)
|
---|
| 19 | 5. [Questions](#questions)
|
---|
| 20 | 6. [Answers](#answers)
|
---|
| 21 | 7. [Separator](#separator)
|
---|
| 22 | 8. [Prompt Types](#prompt)
|
---|
| 23 | 2. [User Interfaces and Layouts](#layouts)
|
---|
| 24 | 1. [Reactive Interface](#reactive)
|
---|
| 25 | 3. [Support](#support)
|
---|
| 26 | 4. [Known issues](#issues)
|
---|
| 27 | 5. [News](#news)
|
---|
| 28 | 6. [Contributing](#contributing)
|
---|
| 29 | 7. [License](#license)
|
---|
| 30 | 8. [Plugins](#plugins)
|
---|
| 31 |
|
---|
| 32 | ## Goal and Philosophy
|
---|
| 33 |
|
---|
| 34 | **`Inquirer.js`** strives to be an easily embeddable and beautiful command line interface for [Node.js](https://nodejs.org/) (and perhaps the "CLI [Xanadu](https://en.wikipedia.org/wiki/Citizen_Kane)").
|
---|
| 35 |
|
---|
| 36 | **`Inquirer.js`** should ease the process of
|
---|
| 37 |
|
---|
| 38 | - providing _error feedback_
|
---|
| 39 | - _asking questions_
|
---|
| 40 | - _parsing_ input
|
---|
| 41 | - _validating_ answers
|
---|
| 42 | - managing _hierarchical prompts_
|
---|
| 43 |
|
---|
| 44 | > **Note:** **`Inquirer.js`** provides the user interface and the inquiry session flow. If you're searching for a full blown command line program utility, then check out [commander](https://github.com/visionmedia/commander.js), [vorpal](https://github.com/dthree/vorpal) or [args](https://github.com/leo/args).
|
---|
| 45 |
|
---|
| 46 | ## [Documentation](#documentation)
|
---|
| 47 |
|
---|
| 48 | <a name="documentation"></a>
|
---|
| 49 |
|
---|
| 50 | ### Installation
|
---|
| 51 |
|
---|
| 52 | <a name="installation"></a>
|
---|
| 53 |
|
---|
| 54 | ```shell
|
---|
| 55 | npm install inquirer
|
---|
| 56 | ```
|
---|
| 57 |
|
---|
| 58 | ```javascript
|
---|
| 59 | var inquirer = require('inquirer');
|
---|
| 60 | inquirer
|
---|
| 61 | .prompt([
|
---|
| 62 | /* Pass your questions in here */
|
---|
| 63 | ])
|
---|
| 64 | .then((answers) => {
|
---|
| 65 | // Use user feedback for... whatever!!
|
---|
| 66 | })
|
---|
| 67 | .catch((error) => {
|
---|
| 68 | if (error.isTtyError) {
|
---|
| 69 | // Prompt couldn't be rendered in the current environment
|
---|
| 70 | } else {
|
---|
| 71 | // Something else went wrong
|
---|
| 72 | }
|
---|
| 73 | });
|
---|
| 74 | ```
|
---|
| 75 |
|
---|
| 76 | <a name="examples"></a>
|
---|
| 77 |
|
---|
| 78 | ### Examples (Run it and see it)
|
---|
| 79 |
|
---|
| 80 | Check out the [`packages/inquirer/examples/`](https://github.com/SBoudrias/Inquirer.js/tree/master/packages/inquirer/examples) folder for code and interface examples.
|
---|
| 81 |
|
---|
| 82 | ```shell
|
---|
| 83 | node packages/inquirer/examples/pizza.js
|
---|
| 84 | node packages/inquirer/examples/checkbox.js
|
---|
| 85 | # etc...
|
---|
| 86 | ```
|
---|
| 87 |
|
---|
| 88 | ### Methods
|
---|
| 89 |
|
---|
| 90 | <a name="methods"></a>
|
---|
| 91 |
|
---|
| 92 | #### `inquirer.prompt(questions, answers) -> promise`
|
---|
| 93 |
|
---|
| 94 | Launch the prompt interface (inquiry session)
|
---|
| 95 |
|
---|
| 96 | - **questions** (Array) containing [Question Object](#question) (using the [reactive interface](#reactive-interface), you can also pass a `Rx.Observable` instance)
|
---|
| 97 | - **answers** (object) contains values of already answered questions. Inquirer will avoid asking answers already provided here. Defaults `{}`.
|
---|
| 98 | - returns a **Promise**
|
---|
| 99 |
|
---|
| 100 | #### `inquirer.registerPrompt(name, prompt)`
|
---|
| 101 |
|
---|
| 102 | Register prompt plugins under `name`.
|
---|
| 103 |
|
---|
| 104 | - **name** (string) name of the this new prompt. (used for question `type`)
|
---|
| 105 | - **prompt** (object) the prompt object itself (the plugin)
|
---|
| 106 |
|
---|
| 107 | #### `inquirer.createPromptModule() -> prompt function`
|
---|
| 108 |
|
---|
| 109 | Create a self contained inquirer module. If you don't want to affect other libraries that also rely on inquirer when you overwrite or add new prompt types.
|
---|
| 110 |
|
---|
| 111 | ```js
|
---|
| 112 | var prompt = inquirer.createPromptModule();
|
---|
| 113 |
|
---|
| 114 | prompt(questions).then(/* ... */);
|
---|
| 115 | ```
|
---|
| 116 |
|
---|
| 117 | ### Objects
|
---|
| 118 |
|
---|
| 119 | <a name="objects"></a>
|
---|
| 120 |
|
---|
| 121 | #### Question
|
---|
| 122 |
|
---|
| 123 | <a name="questions"></a>
|
---|
| 124 | A question object is a `hash` containing question related values:
|
---|
| 125 |
|
---|
| 126 | - **type**: (String) Type of the prompt. Defaults: `input` - Possible values: `input`, `number`, `confirm`,
|
---|
| 127 | `list`, `rawlist`, `expand`, `checkbox`, `password`, `editor`
|
---|
| 128 | - **name**: (String) The name to use when storing the answer in the answers hash. If the name contains periods, it will define a path in the answers hash.
|
---|
| 129 | - **message**: (String|Function) The question to print. If defined as a function, the first parameter will be the current inquirer session answers. Defaults to the value of `name` (followed by a colon).
|
---|
| 130 | - **default**: (String|Number|Boolean|Array|Function) Default value(s) to use if nothing is entered, or a function that returns the default value(s). If defined as a function, the first parameter will be the current inquirer session answers.
|
---|
| 131 | - **choices**: (Array|Function) Choices array or a function returning a choices array. If defined as a function, the first parameter will be the current inquirer session answers.
|
---|
| 132 | Array values can be simple `numbers`, `strings`, or `objects` containing a `name` (to display in list), a `value` (to save in the answers hash), and a `short` (to display after selection) properties. The choices array can also contain [a `Separator`](#separator).
|
---|
| 133 | - **validate**: (Function) Receive the user input and answers hash. Should return `true` if the value is valid, and an error message (`String`) otherwise. If `false` is returned, a default error message is provided.
|
---|
| 134 | - **filter**: (Function) Receive the user input and answers hash. Returns the filtered value to be used inside the program. The value returned will be added to the _Answers_ hash.
|
---|
| 135 | - **transformer**: (Function) Receive the user input, answers hash and option flags, and return a transformed value to display to the user. The transformation only impacts what is shown while editing. It does not modify the answers hash.
|
---|
| 136 | - **when**: (Function, Boolean) Receive the current user answers hash and should return `true` or `false` depending on whether or not this question should be asked. The value can also be a simple boolean.
|
---|
| 137 | - **pageSize**: (Number) Change the number of lines that will be rendered when using `list`, `rawList`, `expand` or `checkbox`.
|
---|
| 138 | - **prefix**: (String) Change the default _prefix_ message.
|
---|
| 139 | - **suffix**: (String) Change the default _suffix_ message.
|
---|
| 140 | - **askAnswered**: (Boolean) Force to prompt the question if the answer already exists.
|
---|
| 141 | - **loop**: (Boolean) Enable list looping. Defaults: `true`
|
---|
| 142 |
|
---|
| 143 | `default`, `choices`(if defined as functions), `validate`, `filter` and `when` functions can be called asynchronously. Either return a promise or use `this.async()` to get a callback you'll call with the final value.
|
---|
| 144 |
|
---|
| 145 | ```javascript
|
---|
| 146 | {
|
---|
| 147 | /* Preferred way: with promise */
|
---|
| 148 | filter() {
|
---|
| 149 | return new Promise(/* etc... */);
|
---|
| 150 | },
|
---|
| 151 |
|
---|
| 152 | /* Legacy way: with this.async */
|
---|
| 153 | validate: function (input) {
|
---|
| 154 | // Declare function as asynchronous, and save the done callback
|
---|
| 155 | var done = this.async();
|
---|
| 156 |
|
---|
| 157 | // Do async stuff
|
---|
| 158 | setTimeout(function() {
|
---|
| 159 | if (typeof input !== 'number') {
|
---|
| 160 | // Pass the return value in the done callback
|
---|
| 161 | done('You need to provide a number');
|
---|
| 162 | return;
|
---|
| 163 | }
|
---|
| 164 | // Pass the return value in the done callback
|
---|
| 165 | done(null, true);
|
---|
| 166 | }, 3000);
|
---|
| 167 | }
|
---|
| 168 | }
|
---|
| 169 | ```
|
---|
| 170 |
|
---|
| 171 | ### Answers
|
---|
| 172 |
|
---|
| 173 | <a name="answers"></a>
|
---|
| 174 | A key/value hash containing the client answers in each prompt.
|
---|
| 175 |
|
---|
| 176 | - **Key** The `name` property of the _question_ object
|
---|
| 177 | - **Value** (Depends on the prompt)
|
---|
| 178 | - `confirm`: (Boolean)
|
---|
| 179 | - `input` : User input (filtered if `filter` is defined) (String)
|
---|
| 180 | - `number`: User input (filtered if `filter` is defined) (Number)
|
---|
| 181 | - `rawlist`, `list` : Selected choice value (or name if no value specified) (String)
|
---|
| 182 |
|
---|
| 183 | ### Separator
|
---|
| 184 |
|
---|
| 185 | <a name="separator"></a>
|
---|
| 186 | A separator can be added to any `choices` array:
|
---|
| 187 |
|
---|
| 188 | ```
|
---|
| 189 | // In the question object
|
---|
| 190 | choices: [ "Choice A", new inquirer.Separator(), "choice B" ]
|
---|
| 191 |
|
---|
| 192 | // Which'll be displayed this way
|
---|
| 193 | [?] What do you want to do?
|
---|
| 194 | > Order a pizza
|
---|
| 195 | Make a reservation
|
---|
| 196 | --------
|
---|
| 197 | Ask opening hours
|
---|
| 198 | Talk to the receptionist
|
---|
| 199 | ```
|
---|
| 200 |
|
---|
| 201 | The constructor takes a facultative `String` value that'll be use as the separator. If omitted, the separator will be `--------`.
|
---|
| 202 |
|
---|
| 203 | Separator instances have a property `type` equal to `separator`. This should allow tools façading Inquirer interface from detecting separator types in lists.
|
---|
| 204 |
|
---|
| 205 | <a name="prompt"></a>
|
---|
| 206 |
|
---|
| 207 | ### Prompt types
|
---|
| 208 |
|
---|
| 209 | ---
|
---|
| 210 |
|
---|
| 211 | > **Note:**: _allowed options written inside square brackets (`[]`) are optional. Others are required._
|
---|
| 212 |
|
---|
| 213 | #### List - `{type: 'list'}`
|
---|
| 214 |
|
---|
| 215 | Take `type`, `name`, `message`, `choices`[, `default`, `filter`, `loop`] properties. (Note that
|
---|
| 216 | default must be the choice `index` in the array or a choice `value`)
|
---|
| 217 |
|
---|
| 218 | 
|
---|
| 219 |
|
---|
| 220 | ---
|
---|
| 221 |
|
---|
| 222 | #### Raw List - `{type: 'rawlist'}`
|
---|
| 223 |
|
---|
| 224 | Take `type`, `name`, `message`, `choices`[, `default`, `filter`, `loop`] properties. (Note that
|
---|
| 225 | default must be the choice `index` in the array)
|
---|
| 226 |
|
---|
| 227 | 
|
---|
| 228 |
|
---|
| 229 | ---
|
---|
| 230 |
|
---|
| 231 | #### Expand - `{type: 'expand'}`
|
---|
| 232 |
|
---|
| 233 | Take `type`, `name`, `message`, `choices`[, `default`] properties. (Note that
|
---|
| 234 | default must be the choice `index` in the array. If `default` key not provided, then `help` will be used as default choice)
|
---|
| 235 |
|
---|
| 236 | Note that the `choices` object will take an extra parameter called `key` for the `expand` prompt. This parameter must be a single (lowercased) character. The `h` option is added by the prompt and shouldn't be defined by the user.
|
---|
| 237 |
|
---|
| 238 | See `examples/expand.js` for a running example.
|
---|
| 239 |
|
---|
| 240 | 
|
---|
| 241 | 
|
---|
| 242 |
|
---|
| 243 | ---
|
---|
| 244 |
|
---|
| 245 | #### Checkbox - `{type: 'checkbox'}`
|
---|
| 246 |
|
---|
| 247 | Take `type`, `name`, `message`, `choices`[, `filter`, `validate`, `default`, `loop`] properties. `default` is expected to be an Array of the checked choices value.
|
---|
| 248 |
|
---|
| 249 | Choices marked as `{checked: true}` will be checked by default.
|
---|
| 250 |
|
---|
| 251 | Choices whose property `disabled` is truthy will be unselectable. If `disabled` is a string, then the string will be outputted next to the disabled choice, otherwise it'll default to `"Disabled"`. The `disabled` property can also be a synchronous function receiving the current answers as argument and returning a boolean or a string.
|
---|
| 252 |
|
---|
| 253 | 
|
---|
| 254 |
|
---|
| 255 | ---
|
---|
| 256 |
|
---|
| 257 | #### Confirm - `{type: 'confirm'}`
|
---|
| 258 |
|
---|
| 259 | Take `type`, `name`, `message`, [`default`] properties. `default` is expected to be a boolean if used.
|
---|
| 260 |
|
---|
| 261 | 
|
---|
| 262 |
|
---|
| 263 | ---
|
---|
| 264 |
|
---|
| 265 | #### Input - `{type: 'input'}`
|
---|
| 266 |
|
---|
| 267 | Take `type`, `name`, `message`[, `default`, `filter`, `validate`, `transformer`] properties.
|
---|
| 268 |
|
---|
| 269 | 
|
---|
| 270 |
|
---|
| 271 | ---
|
---|
| 272 |
|
---|
| 273 | #### Input - `{type: 'number'}`
|
---|
| 274 |
|
---|
| 275 | Take `type`, `name`, `message`[, `default`, `filter`, `validate`, `transformer`] properties.
|
---|
| 276 |
|
---|
| 277 | ---
|
---|
| 278 |
|
---|
| 279 | #### Password - `{type: 'password'}`
|
---|
| 280 |
|
---|
| 281 | Take `type`, `name`, `message`, `mask`,[, `default`, `filter`, `validate`] properties.
|
---|
| 282 |
|
---|
| 283 | 
|
---|
| 284 |
|
---|
| 285 | ---
|
---|
| 286 |
|
---|
| 287 | Note that `mask` is required to hide the actual user input.
|
---|
| 288 |
|
---|
| 289 | #### Editor - `{type: 'editor'}`
|
---|
| 290 |
|
---|
| 291 | Take `type`, `name`, `message`[, `default`, `filter`, `validate`, `postfix`] properties
|
---|
| 292 |
|
---|
| 293 | Launches an instance of the users preferred editor on a temporary file. Once the user exits their editor, the contents of the temporary file are read in as the result. The editor to use is determined by reading the $VISUAL or $EDITOR environment variables. If neither of those are present, notepad (on Windows) or vim (Linux or Mac) is used.
|
---|
| 294 |
|
---|
| 295 | The `postfix` property is useful if you want to provide an extension.
|
---|
| 296 |
|
---|
| 297 | <a name="layouts"></a>
|
---|
| 298 |
|
---|
| 299 | ### Use in Non-Interactive Environments
|
---|
| 300 |
|
---|
| 301 | `prompt()` requires that it is run in an interactive environment. (I.e. [One where `process.stdin.isTTY` is `true`](https://nodejs.org/docs/latest-v12.x/api/process.html#process_a_note_on_process_i_o)). If `prompt()` is invoked outside of such an environment, then `prompt()` will return a rejected promise with an error. For convenience, the error will have a `isTtyError` property to programmatically indicate the cause.
|
---|
| 302 |
|
---|
| 303 | ## User Interfaces and layouts
|
---|
| 304 |
|
---|
| 305 | Along with the prompts, Inquirer offers some basic text UI.
|
---|
| 306 |
|
---|
| 307 | #### Bottom Bar - `inquirer.ui.BottomBar`
|
---|
| 308 |
|
---|
| 309 | This UI present a fixed text at the bottom of a free text zone. This is useful to keep a message to the bottom of the screen while outputting command outputs on the higher section.
|
---|
| 310 |
|
---|
| 311 | ```javascript
|
---|
| 312 | var ui = new inquirer.ui.BottomBar();
|
---|
| 313 |
|
---|
| 314 | // pipe a Stream to the log zone
|
---|
| 315 | outputStream.pipe(ui.log);
|
---|
| 316 |
|
---|
| 317 | // Or simply write output
|
---|
| 318 | ui.log.write('something just happened.');
|
---|
| 319 | ui.log.write('Almost over, standby!');
|
---|
| 320 |
|
---|
| 321 | // During processing, update the bottom bar content to display a loader
|
---|
| 322 | // or output a progress bar, etc
|
---|
| 323 | ui.updateBottomBar('new bottom bar content');
|
---|
| 324 | ```
|
---|
| 325 |
|
---|
| 326 | <a name="reactive"></a>
|
---|
| 327 |
|
---|
| 328 | ## Reactive interface
|
---|
| 329 |
|
---|
| 330 | Internally, Inquirer uses the [JS reactive extension](https://github.com/ReactiveX/rxjs) to handle events and async flows.
|
---|
| 331 |
|
---|
| 332 | This mean you can take advantage of this feature to provide more advanced flows. For example, you can dynamically add questions to be asked:
|
---|
| 333 |
|
---|
| 334 | ```js
|
---|
| 335 | var prompts = new Rx.Subject();
|
---|
| 336 | inquirer.prompt(prompts);
|
---|
| 337 |
|
---|
| 338 | // At some point in the future, push new questions
|
---|
| 339 | prompts.next({
|
---|
| 340 | /* question... */
|
---|
| 341 | });
|
---|
| 342 | prompts.next({
|
---|
| 343 | /* question... */
|
---|
| 344 | });
|
---|
| 345 |
|
---|
| 346 | // When you're done
|
---|
| 347 | prompts.complete();
|
---|
| 348 | ```
|
---|
| 349 |
|
---|
| 350 | And using the return value `process` property, you can access more fine grained callbacks:
|
---|
| 351 |
|
---|
| 352 | ```js
|
---|
| 353 | inquirer.prompt(prompts).ui.process.subscribe(onEachAnswer, onError, onComplete);
|
---|
| 354 | ```
|
---|
| 355 |
|
---|
| 356 | ## Support (OS Terminals)
|
---|
| 357 |
|
---|
| 358 | <a name="support"></a>
|
---|
| 359 |
|
---|
| 360 | You should expect mostly good support for the CLI below. This does not mean we won't
|
---|
| 361 | look at issues found on other command line - feel free to report any!
|
---|
| 362 |
|
---|
| 363 | - **Mac OS**:
|
---|
| 364 | - Terminal.app
|
---|
| 365 | - iTerm
|
---|
| 366 | - **Windows ([Known issues](#issues))**:
|
---|
| 367 | - [ConEmu](https://conemu.github.io/)
|
---|
| 368 | - cmd.exe
|
---|
| 369 | - Powershell
|
---|
| 370 | - Cygwin
|
---|
| 371 | - **Linux (Ubuntu, openSUSE, Arch Linux, etc)**:
|
---|
| 372 | - gnome-terminal (Terminal GNOME)
|
---|
| 373 | - konsole
|
---|
| 374 |
|
---|
| 375 | ## Known issues
|
---|
| 376 |
|
---|
| 377 | <a name="issues"></a>
|
---|
| 378 |
|
---|
| 379 | Running Inquirer together with network streams in Windows platform inside some terminals can result in process hang.
|
---|
| 380 | Workaround: run inside another terminal.
|
---|
| 381 | Please refer to the https://github.com/nodejs/node/issues/21771
|
---|
| 382 |
|
---|
| 383 | ## News on the march (Release notes)
|
---|
| 384 |
|
---|
| 385 | <a name="news"></a>
|
---|
| 386 |
|
---|
| 387 | Please refer to the [GitHub releases section for the changelog](https://github.com/SBoudrias/Inquirer.js/releases)
|
---|
| 388 |
|
---|
| 389 | ## Contributing
|
---|
| 390 |
|
---|
| 391 | <a name="contributing"></a>
|
---|
| 392 |
|
---|
| 393 | **Unit test**
|
---|
| 394 | Unit test are written in [Mocha](https://mochajs.org/). Please add a unit test for every new feature or bug fix. `npm test` to run the test suite.
|
---|
| 395 |
|
---|
| 396 | **Documentation**
|
---|
| 397 | Add documentation for every API change. Feel free to send typo fixes and better docs!
|
---|
| 398 |
|
---|
| 399 | We're looking to offer good support for multiple prompts and environments. If you want to
|
---|
| 400 | help, we'd like to keep a list of testers for each terminal/OS so we can contact you and
|
---|
| 401 | get feedback before release. Let us know if you want to be added to the list (just tweet
|
---|
| 402 | to [@vaxilart](https://twitter.com/Vaxilart)) or just add your name to [the wiki](https://github.com/SBoudrias/Inquirer.js/wiki/Testers)
|
---|
| 403 |
|
---|
| 404 | ## License
|
---|
| 405 |
|
---|
| 406 | <a name="license"></a>
|
---|
| 407 |
|
---|
| 408 | Copyright (c) 2016 Simon Boudrias (twitter: [@vaxilart](https://twitter.com/Vaxilart))
|
---|
| 409 | Licensed under the MIT license.
|
---|
| 410 |
|
---|
| 411 | ## Plugins
|
---|
| 412 |
|
---|
| 413 | <a name="plugins"></a>
|
---|
| 414 |
|
---|
| 415 | ### Prompts
|
---|
| 416 |
|
---|
| 417 | [**autocomplete**](https://github.com/mokkabonna/inquirer-autocomplete-prompt)<br>
|
---|
| 418 | Presents a list of options as the user types, compatible with other packages such as fuzzy (for search)<br>
|
---|
| 419 | <br>
|
---|
| 420 | 
|
---|
| 421 |
|
---|
| 422 | [**checkbox-plus**](https://github.com/faressoft/inquirer-checkbox-plus-prompt)<br>
|
---|
| 423 | Checkbox list with autocomplete and other additions<br>
|
---|
| 424 | <br>
|
---|
| 425 | 
|
---|
| 426 |
|
---|
| 427 | [**inquirer-date-prompt**](https://github.com/haversnail/inquirer-date-prompt)<br>
|
---|
| 428 | Customizable date/time selector with localization support<br>
|
---|
| 429 | <br>
|
---|
| 430 | 
|
---|
| 431 |
|
---|
| 432 | [**datetime**](https://github.com/DerekTBrown/inquirer-datepicker-prompt)<br>
|
---|
| 433 | Customizable date/time selector using both number pad and arrow keys<br>
|
---|
| 434 | <br>
|
---|
| 435 | 
|
---|
| 436 |
|
---|
| 437 | [**inquirer-select-line**](https://github.com/adam-golab/inquirer-select-line)<br>
|
---|
| 438 | Prompt for selecting index in array where add new element<br>
|
---|
| 439 | <br>
|
---|
| 440 | 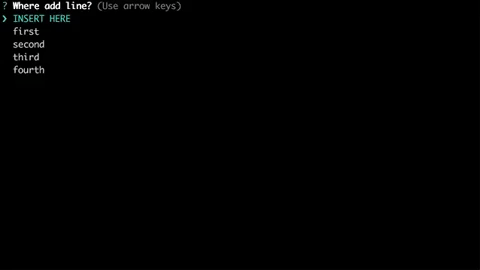
|
---|
| 441 |
|
---|
| 442 | [**command**](https://github.com/sullof/inquirer-command-prompt)<br>
|
---|
| 443 | Simple prompt with command history and dynamic autocomplete<br>
|
---|
| 444 |
|
---|
| 445 | [**inquirer-fuzzy-path**](https://github.com/adelsz/inquirer-fuzzy-path)<br>
|
---|
| 446 | Prompt for fuzzy file/directory selection.<br>
|
---|
| 447 | <br>
|
---|
| 448 | 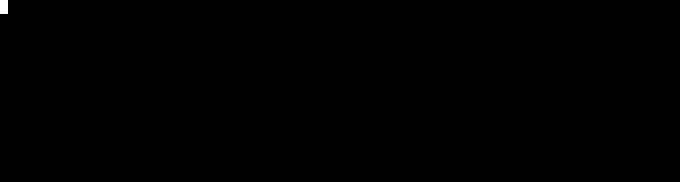
|
---|
| 449 |
|
---|
| 450 | [**inquirer-emoji**](https://github.com/tannerntannern/inquirer-emoji)<br>
|
---|
| 451 | Prompt for inputting emojis.<br>
|
---|
| 452 | <br>
|
---|
| 453 | 
|
---|
| 454 |
|
---|
| 455 | [**inquirer-chalk-pipe**](https://github.com/LitoMore/inquirer-chalk-pipe)<br>
|
---|
| 456 | Prompt for input chalk-pipe style strings<br>
|
---|
| 457 | <br>
|
---|
| 458 | 
|
---|
| 459 |
|
---|
| 460 | [**inquirer-search-checkbox**](https://github.com/clinyong/inquirer-search-checkbox)<br>
|
---|
| 461 | Searchable Inquirer checkbox<br>
|
---|
| 462 |
|
---|
| 463 | [**inquirer-search-list**](https://github.com/robin-rpr/inquirer-search-list)<br>
|
---|
| 464 | Searchable Inquirer list<br>
|
---|
| 465 | <br>
|
---|
| 466 | 
|
---|
| 467 |
|
---|
| 468 | [**inquirer-prompt-suggest**](https://github.com/olistic/inquirer-prompt-suggest)<br>
|
---|
| 469 | Inquirer prompt for your less creative users.<br>
|
---|
| 470 | <br>
|
---|
| 471 | 
|
---|
| 472 |
|
---|
| 473 | [**inquirer-s3**](https://github.com/HQarroum/inquirer-s3)<br>
|
---|
| 474 | An S3 object selector for Inquirer.<br>
|
---|
| 475 | <br>
|
---|
| 476 | 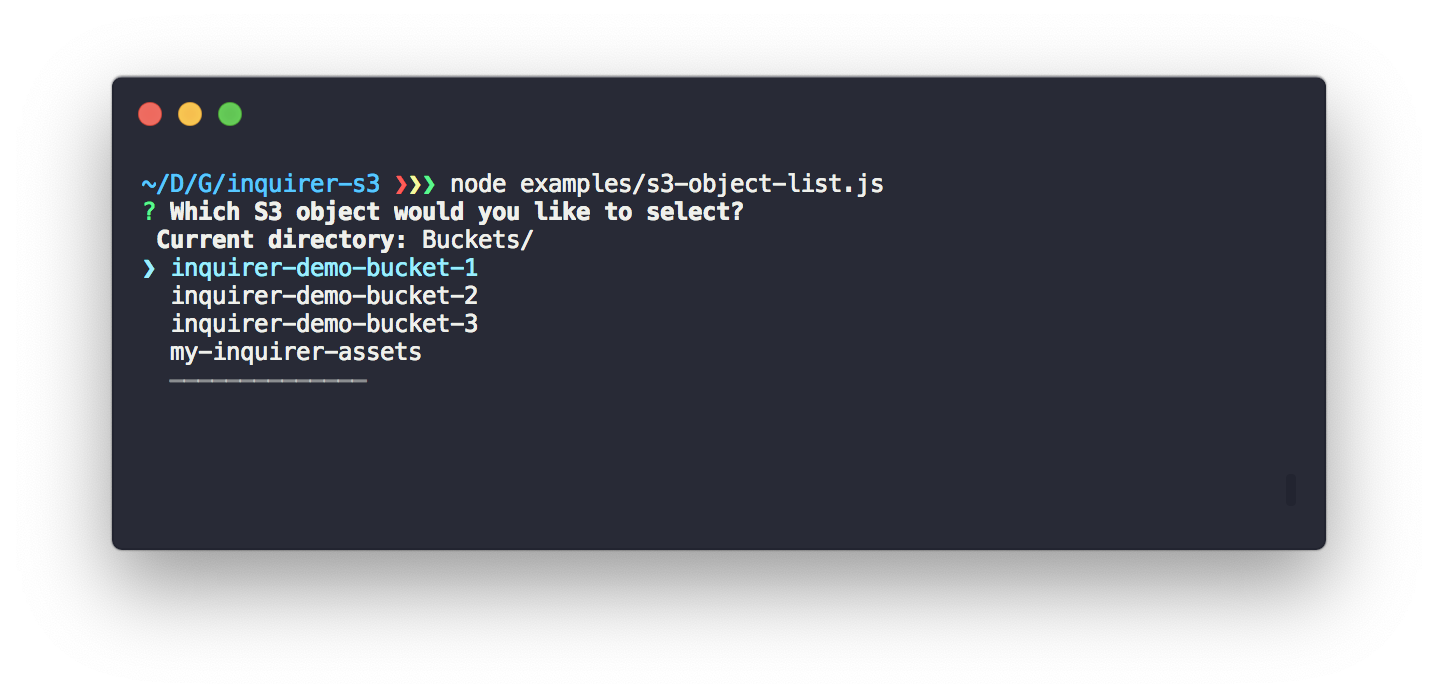
|
---|
| 477 |
|
---|
| 478 | [**inquirer-autosubmit-prompt**](https://github.com/yaodingyd/inquirer-autosubmit-prompt)<br>
|
---|
| 479 | Auto submit based on your current input, saving one extra enter<br>
|
---|
| 480 |
|
---|
| 481 | [**inquirer-file-tree-selection-prompt**](https://github.com/anc95/inquirer-file-tree-selection)<br>
|
---|
| 482 | Inquirer prompt for to select a file or directory in file tree<br>
|
---|
| 483 | <br>
|
---|
| 484 | 
|
---|
| 485 |
|
---|
| 486 | [**inquirer-table-prompt**](https://github.com/eduardoboucas/inquirer-table-prompt)<br>
|
---|
| 487 | A table-like prompt for Inquirer.<br>
|
---|
| 488 | <br>
|
---|
| 489 | 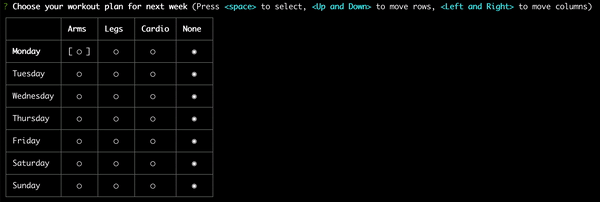
|
---|