1 | # react-inspector
|
---|
2 |
|
---|
3 | [](https://travis-ci.org/storybookjs/react-inspector)
|
---|
4 | [](https://www.npmjs.com/package/react-inspector)
|
---|
5 | [](https://www.npmjs.com/package/react-inspector)
|
---|
6 |
|
---|
7 | Power of [Browser DevTools](https://developers.google.com/web/tools/chrome-devtools/) inspectors right inside your React app. Check out the [interactive playground](https://storybookjs.github.io/react-inspector/) or [storybook](https://react-inspector.netlify.com).
|
---|
8 |
|
---|
9 | 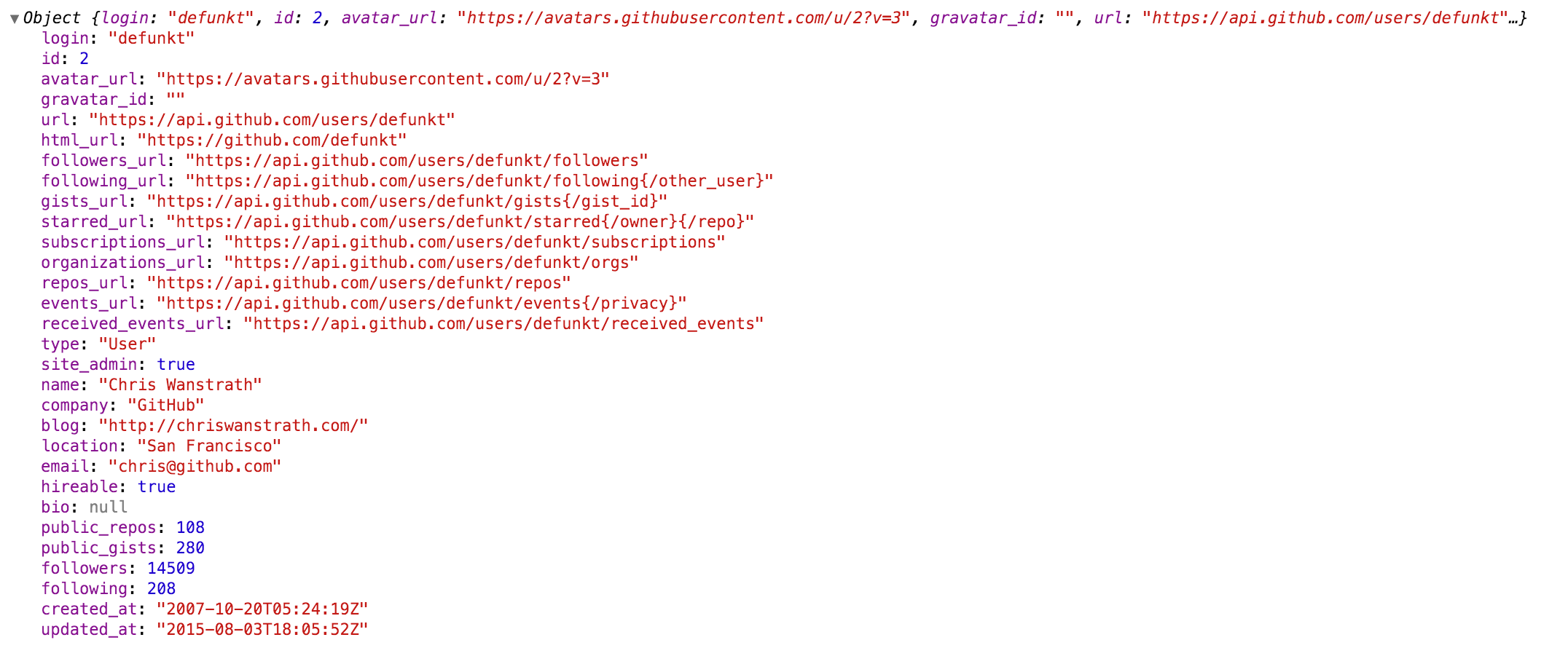
|
---|
10 |
|
---|
11 | 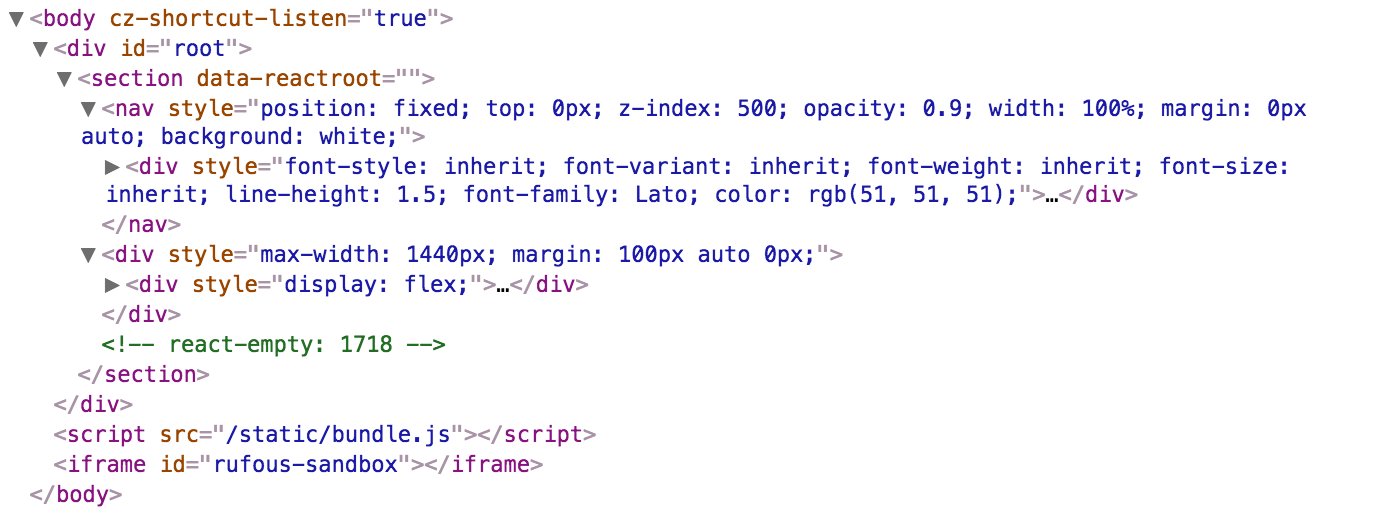
|
---|
12 |
|
---|
13 | 
|
---|
14 |
|
---|
15 | ## Install
|
---|
16 |
|
---|
17 | NPM:
|
---|
18 |
|
---|
19 | ```sh
|
---|
20 | npm install react-inspector
|
---|
21 | ```
|
---|
22 |
|
---|
23 | Recommended versions:
|
---|
24 |
|
---|
25 | - version `3.0.2`: If you are using React 16.8.4 or later.
|
---|
26 | - version `2.3.1`: If you are using an earlier version of React.
|
---|
27 |
|
---|
28 | ## Getting started
|
---|
29 |
|
---|
30 | ### <Inspector />
|
---|
31 |
|
---|
32 | A shorthand for the inspectors.
|
---|
33 |
|
---|
34 | - `<Inspector/>` is equivalent to `<ObjectInspector>` or `<DOMInspector>` if inspecting a DOM Node.
|
---|
35 | - `<Inspector table/>` is equivalent to `<TableInspector>`.
|
---|
36 |
|
---|
37 | ### <ObjectInspector />
|
---|
38 |
|
---|
39 | Like `console.log`. Consider this as a glorified version of `<pre>JSON.stringify(data, null, 2)</pre>`.
|
---|
40 |
|
---|
41 | #### How it works
|
---|
42 |
|
---|
43 | Tree state is saved at root. If you click to expand some elements in the hierarchy, the state will be preserved after the element is unmounted.
|
---|
44 |
|
---|
45 | #### API
|
---|
46 |
|
---|
47 | The component accepts the following props:
|
---|
48 |
|
---|
49 | **`data: PropTypes.any`:** the Javascript object you would like to inspect
|
---|
50 |
|
---|
51 | **`name: PropTypes.string`:** specify the optional name of the root node, default to `undefined`
|
---|
52 |
|
---|
53 | **`expandLevel: PropTypes.number`:** an integer specifying to which level the tree should be initially expanded
|
---|
54 |
|
---|
55 | **`expandPaths: PropTypes.oneOfType([PropTypes.string, PropTypes.array])`:** an array containing all the paths that should be expanded when the component is initialized, or a string of just one path
|
---|
56 |
|
---|
57 | - The path string is similar to [JSONPath](https://goessner.net/articles/JsonPath/).
|
---|
58 | - It is a dot separated string like `$.foo.bar`. `$.foo.bar` expands the path `$.foo.bar` where `$` refers to the root node. Note that it only expands that single node (but not all its parents and the root node). Instead, you should use `expandPaths={['$', '$.foo', '$.foo.bar']}` to expand all the way to the `$.foo.bar` node.
|
---|
59 | - You can refer to array index paths using `['$', '$.1']`
|
---|
60 | - You can use wildcard to expand all paths on a specific level
|
---|
61 | - For example, to expand all first level and second level nodes, use `['$', '$.*']` (equivalent to `expandLevel={2}`)
|
---|
62 | - the results are merged with expandLevel
|
---|
63 |
|
---|
64 | **`showNonenumerable: PropTypes.bool`:** show non-enumerable properties
|
---|
65 |
|
---|
66 | **`sortObjectKeys: PropTypes.oneOfType([PropTypes.bool, PropTypes.func])`:** Sort object keys with optional compare function
|
---|
67 |
|
---|
68 | When `sortObjectKeys={true}` is provided, keys of objects are sorted in alphabetical order except for arrays.
|
---|
69 |
|
---|
70 | **`nodeRenderer: PropTypes.func`:** Use a custom `nodeRenderer` to render the object properties (optional)
|
---|
71 |
|
---|
72 | - Instead of using the default `nodeRenderer`, you can provide a
|
---|
73 | custom function for rendering object properties. The _default_
|
---|
74 | nodeRender looks like this:
|
---|
75 |
|
---|
76 | ```js
|
---|
77 | import { ObjectRootLabel, ObjectLabel } from 'react-inspector'
|
---|
78 |
|
---|
79 | const defaultNodeRenderer = ({ depth, name, data, isNonenumerable, expanded }) =>
|
---|
80 | depth === 0
|
---|
81 | ? <ObjectRootLabel name={name} data={data} />
|
---|
82 | : <ObjectLabel name={name} data={data} isNonenumerable={isNonenumerable} />;
|
---|
83 | ```
|
---|
84 |
|
---|
85 | ### <TableInspector />
|
---|
86 |
|
---|
87 | Like `console.table`.
|
---|
88 |
|
---|
89 | #### API
|
---|
90 |
|
---|
91 | The component accepts the following props:
|
---|
92 |
|
---|
93 | **`data: PropTypes.oneOfType([PropTypes.array, PropTypes.object])`:** the Javascript object you would like to inspect, either an array or an object
|
---|
94 |
|
---|
95 | **`columns: PropTypes.array`:** An array of the names of the columns you'd like to display in the table
|
---|
96 |
|
---|
97 | ### <DOMInspector />
|
---|
98 |
|
---|
99 | #### API
|
---|
100 |
|
---|
101 | The component accepts the following props:
|
---|
102 |
|
---|
103 | **`data: PropTypes.object`:** the DOM Node you would like to inspect
|
---|
104 |
|
---|
105 | #### Usage
|
---|
106 |
|
---|
107 | ```js
|
---|
108 | import { ObjectInspector, TableInspector } from 'react-inspector';
|
---|
109 |
|
---|
110 | // or use the shorthand
|
---|
111 | import { Inspector } from 'react-inspector';
|
---|
112 |
|
---|
113 | const MyComponent = ({ data }) =>
|
---|
114 | <div>
|
---|
115 | <ObjectInspector data={data} />
|
---|
116 | <TableInspector data={data} />
|
---|
117 |
|
---|
118 | <Inspector data={data} />
|
---|
119 | <Inspector table data={data} />
|
---|
120 | </div>
|
---|
121 |
|
---|
122 | let data = { /* ... */ };
|
---|
123 |
|
---|
124 | ReactDOM.render(
|
---|
125 | <MyComponent data={data} />,
|
---|
126 | document.getElementById('root')
|
---|
127 | );
|
---|
128 | ```
|
---|
129 |
|
---|
130 | Try embedding the inspectors inside a component's render() method to provide a live view for its props/state (Works even better with hot reloading).
|
---|
131 |
|
---|
132 | ### More Examples
|
---|
133 |
|
---|
134 | Check out the storybook for more examples.
|
---|
135 |
|
---|
136 | ```sh
|
---|
137 | npm install && npm run storybook
|
---|
138 | ```
|
---|
139 |
|
---|
140 | Open [http://localhost:9001/](http://localhost:9001/)
|
---|
141 |
|
---|
142 | ## Theme
|
---|
143 |
|
---|
144 | By specifying the `theme` prop you can customize the inspectors. `theme` prop can be
|
---|
145 |
|
---|
146 | 1. a string referring to a preset theme (`"chromeLight"` or `"chromeDark"`, default to `"chromeLight"`)
|
---|
147 | 2. or a custom object that provides the necessary variables. Checkout [`src/styles/themes`](https://github.com/storybookjs/react-inspector/tree/master/src/styles/themes) for possible theme variables.
|
---|
148 |
|
---|
149 | **Example 1:** Using a preset theme:
|
---|
150 |
|
---|
151 | ```js
|
---|
152 | <Inspector theme="chromeDark" data={{a: 'a', b: 'b'}}/>
|
---|
153 | ```
|
---|
154 |
|
---|
155 | **Example 2:** changing the tree node indentation by inheriting the chrome light theme:
|
---|
156 |
|
---|
157 | ```js
|
---|
158 | import { chromeLight } from 'react-inspector'
|
---|
159 |
|
---|
160 | <Inspector theme={{...chromeLight, ...({ TREENODE_PADDING_LEFT: 20 })}} data={{a: 'a', b: 'b'}}/>
|
---|
161 | ```
|
---|
162 |
|
---|
163 | ## Roadmap
|
---|
164 |
|
---|
165 | Type of inspectors:
|
---|
166 |
|
---|
167 | - [x] Tree style
|
---|
168 | - [x] common objects
|
---|
169 | - [x] DOM nodes
|
---|
170 | - [x] Table style
|
---|
171 | - [ ] Column resizer
|
---|
172 | - [ ] Group style
|
---|
173 |
|
---|
174 | ## Contribution
|
---|
175 |
|
---|
176 | Contribution is welcome. [Past contributors](https://github.com/storybookjs/react-inspector/graphs/contributors)
|
---|
177 |
|
---|
178 | ## Additional
|
---|
179 |
|
---|
180 | - If you intend to capture `console.log`s, you may want to look at [`console-feed`](https://www.npmjs.com/package/console-feed).
|
---|
181 | - `react-object-inspector` package will be deprecated. `<ObjectInspector/>` is now part of the new package `react-inspector`.
|
---|
182 | - Why inline style? [This document](https://github.com/erikras/react-redux-universal-hot-example/blob/master/docs/InlineStyles.md) summarizes it well.
|
---|