[2aea0fd] | 1 | # PostgreSQL ASP.NET 7
|
---|
| 2 |
|
---|
| 3 | Convert an ASP.NET Core Web Application project to use PostgreSQL with Entity Framework.
|
---|
| 4 |
|
---|
| 5 | This enables development of ASP.NET Core projects using [VS Code](https://code.visualstudio.com/) on macOS or linux targets.
|
---|
| 6 |
|
---|
| 7 | This project uses [.NET 7.0](https://dotnet.microsoft.com/en-us/download/dotnet/7.0) target framework, ASP.NET Core Web Application MVC project scaffold from Visual Studio 2022 (version 17.4).
|
---|
| 8 |
|
---|
| 9 | 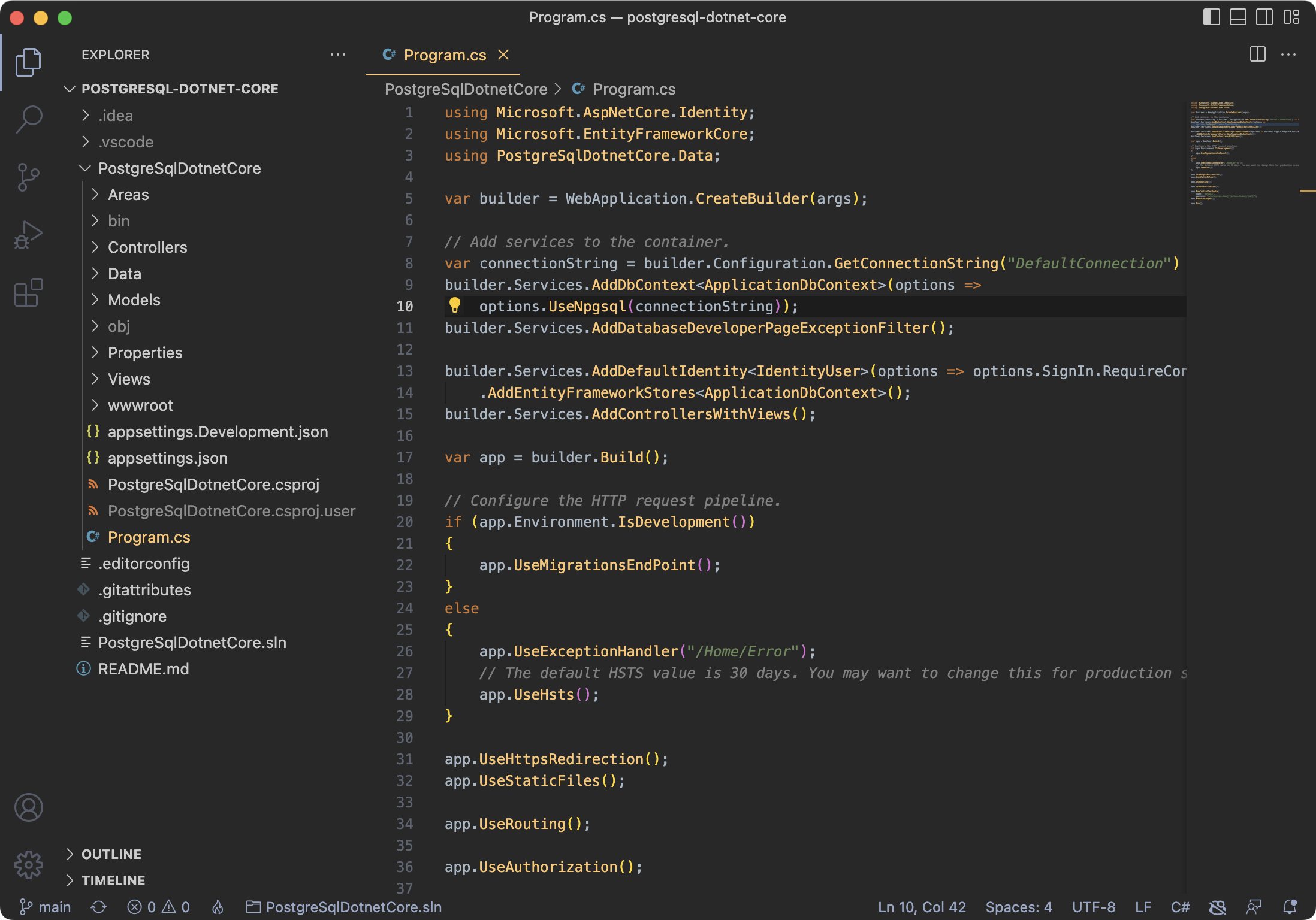
|
---|
| 10 |
|
---|
| 11 | Project setup has already been completed in this repository - assure [environment setup](#environment-setup); then, jump to [running the solution](#running-the-solution).
|
---|
| 12 |
|
---|
| 13 |
|
---|
| 14 | ## Environment Setup
|
---|
| 15 |
|
---|
| 16 | This project requires PostgreSQL - installation instructions are provided below.
|
---|
| 17 |
|
---|
| 18 | If using Visual Studio Code, you will need to generate ASP.NET Core developer certificates by issuing the following commands from a terminal:
|
---|
| 19 |
|
---|
| 20 | dotnet dev-certs https --clean
|
---|
| 21 | dotnet dev-certs https
|
---|
| 22 |
|
---|
| 23 | For command line `database ef` commands, you will need to install Entity Framework Core tools .NET CLI:
|
---|
| 24 |
|
---|
| 25 | dotnet tool install --global dotnet-ef
|
---|
| 26 |
|
---|
| 27 |
|
---|
| 28 | ## Project Setup
|
---|
| 29 |
|
---|
| 30 |
|
---|
| 31 | Below, instructions are referenced to use PostgreSQL in a ASP.NET Core project.
|
---|
| 32 |
|
---|
| 33 |
|
---|
| 34 | ### Install NuGet packages
|
---|
| 35 |
|
---|
| 36 | Install the `Npgsql.EntityFrameworkCore.PostgreSQL` NuGet package in the ASP.NET web application.
|
---|
| 37 |
|
---|
| 38 | To do this, you can use the `dotnet` command line by executing:
|
---|
| 39 |
|
---|
| 40 | $ dotnet add package Npgsql.EntityFrameworkCore.PostgreSQL --version 3.1.2
|
---|
| 41 |
|
---|
| 42 | Or, edit the project's .csproj file and add the following line in the `PackageReference` item group:
|
---|
| 43 |
|
---|
| 44 | ```xml
|
---|
| 45 | <PackageReference Include="Npgsql.EntityFrameworkCore.PostgreSQL" Version="3.1.2" />
|
---|
| 46 | ```
|
---|
| 47 |
|
---|
| 48 |
|
---|
| 49 | ### Update appsettings.json
|
---|
| 50 |
|
---|
| 51 | Configure connection string in project's appsettings.json, replacing the `username`, `password`, and `dbname` appropriately:
|
---|
| 52 |
|
---|
| 53 | ```json
|
---|
| 54 | "ConnectionStrings": {
|
---|
| 55 | "DefaultConnection": "User ID=username;Password=password;Server=localhost;Port=5432;Database=dbname;Integrated Security=true;Pooling=true;"
|
---|
| 56 | },
|
---|
| 57 | ```
|
---|
| 58 |
|
---|
| 59 |
|
---|
| 60 | ### Modify Program.cs
|
---|
| 61 |
|
---|
| 62 | Inside Program.cs replace the `UseSqlServer` options with `UseNpgsql`:
|
---|
| 63 |
|
---|
| 64 | ```cs
|
---|
| 65 | builder.Services.AddDbContext<ApplicationDbContext>(options =>
|
---|
| 66 | options.UseNpgsql(connectionString));
|
---|
| 67 | ```
|
---|
| 68 |
|
---|
| 69 |
|
---|
| 70 | ## Running the solution
|
---|
| 71 |
|
---|
| 72 | Before the solution can be executed, be sure to run entity framework migrations.
|
---|
| 73 |
|
---|
| 74 |
|
---|
| 75 | ### Migration Issues with DbContext
|
---|
| 76 |
|
---|
| 77 | Initial migrations may fail, due to ASP.NET Core template come with a pre-generation migration for SQL Server.
|
---|
| 78 |
|
---|
| 79 | When trying to run the migration, you might see errors such as:
|
---|
| 80 | > Npgsql.PostgresException (0x80004005): 42704: type "nvarchar" does not exist
|
---|
| 81 | >
|
---|
| 82 | > System.NullReferenceException: Object reference not set to an instance of an object.
|
---|
| 83 | >
|
---|
| 84 | > System.InvalidOperationException: No mapping to a relational type can be found for property 'Microsoft.AspNetCore.Identity.IdentityUser.TwoFactorEnabled' with the CLR type 'bool'.
|
---|
| 85 |
|
---|
| 86 | Delete the entire Migrations folder, and regenerate new inital migrations.
|
---|
| 87 |
|
---|
| 88 | Generate a new migration using Visual Studio Package Manager Console (from menu: Tools -> NuGet Package Manager -> Package Manager Console):
|
---|
| 89 |
|
---|
| 90 | PM> Add-Migration
|
---|
| 91 |
|
---|
| 92 | Or, from the command line via DotNet CLI:
|
---|
| 93 |
|
---|
| 94 | $ dotnet ef migrations add Initial
|
---|
| 95 |
|
---|
| 96 | If dotnet migration tools don't exist, remember to install the tools using the instruction above in the [environment setup](#environment-setup).
|
---|
| 97 |
|
---|
| 98 |
|
---|
| 99 | ### Run Entity Framework Migrations
|
---|
| 100 |
|
---|
| 101 | Execute the migration using either Visual Studio Package Manager Console (from menu: Tools -> NuGet Package Manager -> Package Manager Console):
|
---|
| 102 |
|
---|
| 103 | PM> Update-Database
|
---|
| 104 |
|
---|
| 105 | Or, from the command line via DotNet CLI, execute the following command inside the project directory, **where the .csproj file is located**:
|
---|
| 106 |
|
---|
| 107 | $ dotnet ef database update
|
---|
| 108 |
|
---|
| 109 | After running the migration, the database is created and web application is ready to be run.
|
---|
| 110 |
|
---|
| 111 |
|
---|
| 112 | ## Setting up a PostgresSQL server on Mac
|
---|
| 113 |
|
---|
| 114 | Here are instructions to setup a PostgreSQL server on Mac using Homebrew.
|
---|
| 115 |
|
---|
| 116 |
|
---|
| 117 | ### Installing PostgreSQL on Mac
|
---|
| 118 |
|
---|
| 119 | Use [brew](https://brew.sh/) to install PostgreSQL, then launch the service:
|
---|
| 120 |
|
---|
| 121 | $ brew install postgresql
|
---|
| 122 | $ brew services start postgresql
|
---|
| 123 |
|
---|
| 124 |
|
---|
| 125 | ### Create a user
|
---|
| 126 |
|
---|
| 127 | Create a user using the `createuser` command from a terminal, where `username` is your desired new user name. Using the `-P` argument, you will be prompted to setup a password.
|
---|
| 128 |
|
---|
| 129 | $ createuser username -P
|
---|
| 130 |
|
---|
| 131 |
|
---|
| 132 | ### Create a database
|
---|
| 133 |
|
---|
| 134 | Create your database using the `createdb` command from a terminal, where `dbname` is your desired new database name.
|
---|
| 135 |
|
---|
| 136 | $ createdb dbname
|
---|
| 137 |
|
---|
| 138 | At this time, run the solution's Entity Framework migrations (see above for instructions).
|
---|
| 139 |
|
---|
| 140 |
|
---|
| 141 | ### Verifying database
|
---|
| 142 |
|
---|
| 143 | Launch PostgreSQL interactive terminal and connect to the database.
|
---|
| 144 |
|
---|
| 145 | $ psql dbname
|
---|
| 146 |
|
---|
| 147 |
|
---|
| 148 | From the PostgreSQL interface terminal, List tables using the `\dt` command:
|
---|
| 149 |
|
---|
| 150 | dbname=# \dt
|
---|
| 151 | List of relations
|
---|
| 152 | Schema | Name | Type | Owner
|
---|
| 153 | --------+-----------------------+-------+--------------
|
---|
| 154 | public | AspNetRoleClaims | table | username
|
---|
| 155 | public | AspNetRoles | table | username
|
---|
| 156 | public | AspNetUserClaims | table | username
|
---|
| 157 | public | AspNetUserLogins | table | username
|
---|
| 158 | public | AspNetUserRoles | table | username
|
---|
| 159 | public | AspNetUserTokens | table | username
|
---|
| 160 | public | AspNetUsers | table | username
|
---|
| 161 | public | __EFMigrationsHistory | table | username
|
---|
| 162 | (8 rows)
|
---|
| 163 |
|
---|
| 164 |
|
---|
| 165 | ### Database permissions issues
|
---|
| 166 |
|
---|
| 167 | If permissions were not setup properly during the creation of the database, retroactively fix by granting privileges where `dbname` is your database name and `username` is the user you created:
|
---|
| 168 |
|
---|
| 169 | $ psql dbname
|
---|
| 170 | dbname=# GRANT ALL PRIVILEGES ON ALL TABLES IN SCHEMA public TO username;
|
---|