1 | # fastq
|
---|
2 |
|
---|
3 | ![ci][ci-url]
|
---|
4 | [![npm version][npm-badge]][npm-url]
|
---|
5 |
|
---|
6 | Fast, in memory work queue.
|
---|
7 |
|
---|
8 | Benchmarks (1 million tasks):
|
---|
9 |
|
---|
10 | * setImmediate: 812ms
|
---|
11 | * fastq: 854ms
|
---|
12 | * async.queue: 1298ms
|
---|
13 | * neoAsync.queue: 1249ms
|
---|
14 |
|
---|
15 | Obtained on node 12.16.1, on a dedicated server.
|
---|
16 |
|
---|
17 | If you need zero-overhead series function call, check out
|
---|
18 | [fastseries](http://npm.im/fastseries). For zero-overhead parallel
|
---|
19 | function call, check out [fastparallel](http://npm.im/fastparallel).
|
---|
20 |
|
---|
21 | [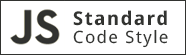](https://github.com/feross/standard)
|
---|
22 |
|
---|
23 | * <a href="#install">Installation</a>
|
---|
24 | * <a href="#usage">Usage</a>
|
---|
25 | * <a href="#api">API</a>
|
---|
26 | * <a href="#license">Licence & copyright</a>
|
---|
27 |
|
---|
28 | ## Install
|
---|
29 |
|
---|
30 | `npm i fastq --save`
|
---|
31 |
|
---|
32 | ## Usage (callback API)
|
---|
33 |
|
---|
34 | ```js
|
---|
35 | 'use strict'
|
---|
36 |
|
---|
37 | const queue = require('fastq')(worker, 1)
|
---|
38 |
|
---|
39 | queue.push(42, function (err, result) {
|
---|
40 | if (err) { throw err }
|
---|
41 | console.log('the result is', result)
|
---|
42 | })
|
---|
43 |
|
---|
44 | function worker (arg, cb) {
|
---|
45 | cb(null, arg * 2)
|
---|
46 | }
|
---|
47 | ```
|
---|
48 |
|
---|
49 | ## Usage (promise API)
|
---|
50 |
|
---|
51 | ```js
|
---|
52 | const queue = require('fastq').promise(worker, 1)
|
---|
53 |
|
---|
54 | async function worker (arg) {
|
---|
55 | return arg * 2
|
---|
56 | }
|
---|
57 |
|
---|
58 | async function run () {
|
---|
59 | const result = await queue.push(42)
|
---|
60 | console.log('the result is', result)
|
---|
61 | }
|
---|
62 |
|
---|
63 | run()
|
---|
64 | ```
|
---|
65 |
|
---|
66 | ### Setting "this"
|
---|
67 |
|
---|
68 | ```js
|
---|
69 | 'use strict'
|
---|
70 |
|
---|
71 | const that = { hello: 'world' }
|
---|
72 | const queue = require('fastq')(that, worker, 1)
|
---|
73 |
|
---|
74 | queue.push(42, function (err, result) {
|
---|
75 | if (err) { throw err }
|
---|
76 | console.log(this)
|
---|
77 | console.log('the result is', result)
|
---|
78 | })
|
---|
79 |
|
---|
80 | function worker (arg, cb) {
|
---|
81 | console.log(this)
|
---|
82 | cb(null, arg * 2)
|
---|
83 | }
|
---|
84 | ```
|
---|
85 |
|
---|
86 | ### Using with TypeScript (callback API)
|
---|
87 |
|
---|
88 | ```ts
|
---|
89 | 'use strict'
|
---|
90 |
|
---|
91 | import * as fastq from "fastq";
|
---|
92 | import type { queue, done } from "fastq";
|
---|
93 |
|
---|
94 | type Task = {
|
---|
95 | id: number
|
---|
96 | }
|
---|
97 |
|
---|
98 | const q: queue<Task> = fastq(worker, 1)
|
---|
99 |
|
---|
100 | q.push({ id: 42})
|
---|
101 |
|
---|
102 | function worker (arg: Task, cb: done) {
|
---|
103 | console.log(arg.id)
|
---|
104 | cb(null)
|
---|
105 | }
|
---|
106 | ```
|
---|
107 |
|
---|
108 | ### Using with TypeScript (promise API)
|
---|
109 |
|
---|
110 | ```ts
|
---|
111 | 'use strict'
|
---|
112 |
|
---|
113 | import * as fastq from "fastq";
|
---|
114 | import type { queueAsPromised } from "fastq";
|
---|
115 |
|
---|
116 | type Task = {
|
---|
117 | id: number
|
---|
118 | }
|
---|
119 |
|
---|
120 | const q: queueAsPromised<Task> = fastq.promise(asyncWorker, 1)
|
---|
121 |
|
---|
122 | q.push({ id: 42}).catch((err) => console.error(err))
|
---|
123 |
|
---|
124 | async function asyncWorker (arg: Task): Promise<void> {
|
---|
125 | // No need for a try-catch block, fastq handles errors automatically
|
---|
126 | console.log(arg.id)
|
---|
127 | }
|
---|
128 | ```
|
---|
129 |
|
---|
130 | ## API
|
---|
131 |
|
---|
132 | * <a href="#fastqueue"><code>fastqueue()</code></a>
|
---|
133 | * <a href="#push"><code>queue#<b>push()</b></code></a>
|
---|
134 | * <a href="#unshift"><code>queue#<b>unshift()</b></code></a>
|
---|
135 | * <a href="#pause"><code>queue#<b>pause()</b></code></a>
|
---|
136 | * <a href="#resume"><code>queue#<b>resume()</b></code></a>
|
---|
137 | * <a href="#idle"><code>queue#<b>idle()</b></code></a>
|
---|
138 | * <a href="#length"><code>queue#<b>length()</b></code></a>
|
---|
139 | * <a href="#getQueue"><code>queue#<b>getQueue()</b></code></a>
|
---|
140 | * <a href="#kill"><code>queue#<b>kill()</b></code></a>
|
---|
141 | * <a href="#killAndDrain"><code>queue#<b>killAndDrain()</b></code></a>
|
---|
142 | * <a href="#error"><code>queue#<b>error()</b></code></a>
|
---|
143 | * <a href="#concurrency"><code>queue#<b>concurrency</b></code></a>
|
---|
144 | * <a href="#drain"><code>queue#<b>drain</b></code></a>
|
---|
145 | * <a href="#empty"><code>queue#<b>empty</b></code></a>
|
---|
146 | * <a href="#saturated"><code>queue#<b>saturated</b></code></a>
|
---|
147 | * <a href="#promise"><code>fastqueue.promise()</code></a>
|
---|
148 |
|
---|
149 | -------------------------------------------------------
|
---|
150 | <a name="fastqueue"></a>
|
---|
151 | ### fastqueue([that], worker, concurrency)
|
---|
152 |
|
---|
153 | Creates a new queue.
|
---|
154 |
|
---|
155 | Arguments:
|
---|
156 |
|
---|
157 | * `that`, optional context of the `worker` function.
|
---|
158 | * `worker`, worker function, it would be called with `that` as `this`,
|
---|
159 | if that is specified.
|
---|
160 | * `concurrency`, number of concurrent tasks that could be executed in
|
---|
161 | parallel.
|
---|
162 |
|
---|
163 | -------------------------------------------------------
|
---|
164 | <a name="push"></a>
|
---|
165 | ### queue.push(task, done)
|
---|
166 |
|
---|
167 | Add a task at the end of the queue. `done(err, result)` will be called
|
---|
168 | when the task was processed.
|
---|
169 |
|
---|
170 | -------------------------------------------------------
|
---|
171 | <a name="unshift"></a>
|
---|
172 | ### queue.unshift(task, done)
|
---|
173 |
|
---|
174 | Add a task at the beginning of the queue. `done(err, result)` will be called
|
---|
175 | when the task was processed.
|
---|
176 |
|
---|
177 | -------------------------------------------------------
|
---|
178 | <a name="pause"></a>
|
---|
179 | ### queue.pause()
|
---|
180 |
|
---|
181 | Pause the processing of tasks. Currently worked tasks are not
|
---|
182 | stopped.
|
---|
183 |
|
---|
184 | -------------------------------------------------------
|
---|
185 | <a name="resume"></a>
|
---|
186 | ### queue.resume()
|
---|
187 |
|
---|
188 | Resume the processing of tasks.
|
---|
189 |
|
---|
190 | -------------------------------------------------------
|
---|
191 | <a name="idle"></a>
|
---|
192 | ### queue.idle()
|
---|
193 |
|
---|
194 | Returns `false` if there are tasks being processed or waiting to be processed.
|
---|
195 | `true` otherwise.
|
---|
196 |
|
---|
197 | -------------------------------------------------------
|
---|
198 | <a name="length"></a>
|
---|
199 | ### queue.length()
|
---|
200 |
|
---|
201 | Returns the number of tasks waiting to be processed (in the queue).
|
---|
202 |
|
---|
203 | -------------------------------------------------------
|
---|
204 | <a name="getQueue"></a>
|
---|
205 | ### queue.getQueue()
|
---|
206 |
|
---|
207 | Returns all the tasks be processed (in the queue). Returns empty array when there are no tasks
|
---|
208 |
|
---|
209 | -------------------------------------------------------
|
---|
210 | <a name="kill"></a>
|
---|
211 | ### queue.kill()
|
---|
212 |
|
---|
213 | Removes all tasks waiting to be processed, and reset `drain` to an empty
|
---|
214 | function.
|
---|
215 |
|
---|
216 | -------------------------------------------------------
|
---|
217 | <a name="killAndDrain"></a>
|
---|
218 | ### queue.killAndDrain()
|
---|
219 |
|
---|
220 | Same than `kill` but the `drain` function will be called before reset to empty.
|
---|
221 |
|
---|
222 | -------------------------------------------------------
|
---|
223 | <a name="error"></a>
|
---|
224 | ### queue.error(handler)
|
---|
225 |
|
---|
226 | Set a global error handler. `handler(err, task)` will be called
|
---|
227 | each time a task is completed, `err` will be not null if the task has thrown an error.
|
---|
228 |
|
---|
229 | -------------------------------------------------------
|
---|
230 | <a name="concurrency"></a>
|
---|
231 | ### queue.concurrency
|
---|
232 |
|
---|
233 | Property that returns the number of concurrent tasks that could be executed in
|
---|
234 | parallel. It can be altered at runtime.
|
---|
235 |
|
---|
236 | -------------------------------------------------------
|
---|
237 | <a name="drain"></a>
|
---|
238 | ### queue.drain
|
---|
239 |
|
---|
240 | Function that will be called when the last
|
---|
241 | item from the queue has been processed by a worker.
|
---|
242 | It can be altered at runtime.
|
---|
243 |
|
---|
244 | -------------------------------------------------------
|
---|
245 | <a name="empty"></a>
|
---|
246 | ### queue.empty
|
---|
247 |
|
---|
248 | Function that will be called when the last
|
---|
249 | item from the queue has been assigned to a worker.
|
---|
250 | It can be altered at runtime.
|
---|
251 |
|
---|
252 | -------------------------------------------------------
|
---|
253 | <a name="saturated"></a>
|
---|
254 | ### queue.saturated
|
---|
255 |
|
---|
256 | Function that will be called when the queue hits the concurrency
|
---|
257 | limit.
|
---|
258 | It can be altered at runtime.
|
---|
259 |
|
---|
260 | -------------------------------------------------------
|
---|
261 | <a name="promise"></a>
|
---|
262 | ### fastqueue.promise([that], worker(arg), concurrency)
|
---|
263 |
|
---|
264 | Creates a new queue with `Promise` apis. It also offers all the methods
|
---|
265 | and properties of the object returned by [`fastqueue`](#fastqueue) with the modified
|
---|
266 | [`push`](#pushPromise) and [`unshift`](#unshiftPromise) methods.
|
---|
267 |
|
---|
268 | Node v10+ is required to use the promisified version.
|
---|
269 |
|
---|
270 | Arguments:
|
---|
271 | * `that`, optional context of the `worker` function.
|
---|
272 | * `worker`, worker function, it would be called with `that` as `this`,
|
---|
273 | if that is specified. It MUST return a `Promise`.
|
---|
274 | * `concurrency`, number of concurrent tasks that could be executed in
|
---|
275 | parallel.
|
---|
276 |
|
---|
277 | <a name="pushPromise"></a>
|
---|
278 | #### queue.push(task) => Promise
|
---|
279 |
|
---|
280 | Add a task at the end of the queue. The returned `Promise` will be fulfilled (rejected)
|
---|
281 | when the task is completed successfully (unsuccessfully).
|
---|
282 |
|
---|
283 | This promise could be ignored as it will not lead to a `'unhandledRejection'`.
|
---|
284 |
|
---|
285 | <a name="unshiftPromise"></a>
|
---|
286 | #### queue.unshift(task) => Promise
|
---|
287 |
|
---|
288 | Add a task at the beginning of the queue. The returned `Promise` will be fulfilled (rejected)
|
---|
289 | when the task is completed successfully (unsuccessfully).
|
---|
290 |
|
---|
291 | This promise could be ignored as it will not lead to a `'unhandledRejection'`.
|
---|
292 |
|
---|
293 | <a name="drained"></a>
|
---|
294 | #### queue.drained() => Promise
|
---|
295 |
|
---|
296 | Wait for the queue to be drained. The returned `Promise` will be resolved when all tasks in the queue have been processed by a worker.
|
---|
297 |
|
---|
298 | This promise could be ignored as it will not lead to a `'unhandledRejection'`.
|
---|
299 |
|
---|
300 | ## License
|
---|
301 |
|
---|
302 | ISC
|
---|
303 |
|
---|
304 | [ci-url]: https://github.com/mcollina/fastq/workflows/ci/badge.svg
|
---|
305 | [npm-badge]: https://badge.fury.io/js/fastq.svg
|
---|
306 | [npm-url]: https://badge.fury.io/js/fastq
|
---|